Last updated on October 8th, 2023 at 03:20 pm

Arrays are one of the most useful data structures used in programming. They allow us to enter and process blocks of data of the same type together.
In programming, and specifically in Pascal, arrays are generally used with loops to process repetitive data, reducing the number of lines of code. In this article, we will look at the following:
- What arrays are;
- The purpose of arrays;
- How to declare arrays;
- Writing pseudocodes of arrays; and
- An example of an array in the Pascal programming language.
As an added bonus, we also use control structures and the for loop and while loops in a more intricate way. To read our introduction to for loops, visit for and while loop in Pascal programming.
What are arrays
An array is a combination of memory locations in series with a single variable name used to store a collection of data with the same data type. For instance, if you need to store the ages of students in a class, you may use an array called ages to store the ages of all the students. Each piece of data is stored and retrieved using an index number.
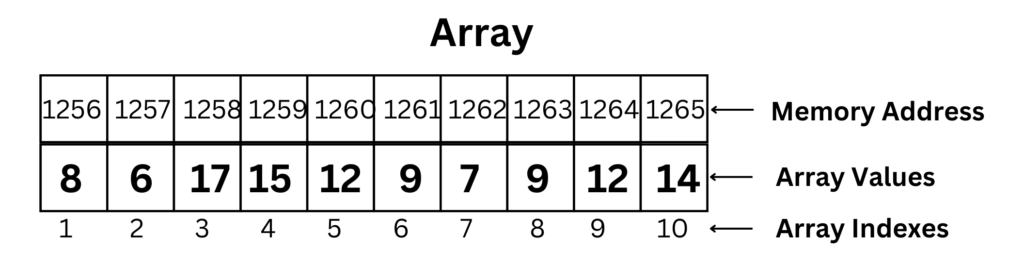
What are arrays used for?
Let’s imagine that you were asked to write a program that would take in the names of 100 students, order them alphabetically, and print this as a list on the screen.
Arrays allow us to store all of the names in one place rather than having to declare and store data in many different variables. We can store and sort the names of all of these students in a single array and then print them on the screen.
How to declare an arrays – Understanding an array declaration
Unlike other types of variables, when you declare an array, you may specify the number of items that will be stored in it. In Pascal programming, we declare arrays like this:
nameF : array[0..4] of string;
The first part, nameF, is the name of the variable. The second part, array[0..4], indicates that it is an array with 5 places (0 to 4) to accommodate data. The last part, string, indicates the type of data that will be stored in the array.
Example 1 – Arrays
Write a program that takes the names and gender of 10 students in a class. The program should separate the students by gender and print the names on the screen.
Input | Process | Output |
---|---|---|
names | 1. Read the name of each child. | gender |
gender | 2. Read the gender of each child. | names |
3. Separate the students by gender. | ||
4. Print the students’ names and gender . |
Algorithm of example 1 – Looping with arrays
Algorithm sex;
start
- Count = 0
- countM = 0
- countF = 0
- i = 0
- While count < 4
- start
- a. Display: Please enter the gender;
- b. read gender
- c. if(gender = f)
- start
- i. Display: Please enter the name of the girl.
- ii. Read: nameF.
- iii. Increment countF
- stop
- d. else
- start
- i. Display: Please enter the boy’s name.
- iii. Read: nameM[countM]
- iii. increment countM
- stop
- vi. count = count + 1
- Stop
- if(countM>=0)
- start
- i. Display: The boys are:
- ii. for i =1 to countM
- start
- 1. countM = countM – 1
- 2. Display nameM[countM]
- stop
- stop
- if(countF>=0)
- start
- a. for i = 1 to countF
- start
- i. countF = countF – 1
- ii. Display: nameF(countF)
- stop
- stop
stop
Pascal program using Arrays
Program sex;
var
count : integer;
student : string;
gender : char;
nameF : array[0..4] of string;
nameM : array[0..4] of string;
countM : integer;
countF : integer;
i : integer;
begin
count := 0;
countM := 0;
countF := 0;
i:=0;
while(count<4)do
begin
writeln('Please enter the gender:');
readln(gender);
if(gender = 'f')then
begin
writeln('Please enter the name of the girl: ');
readln(nameF[countF]);
countF := countF + 1;
end
else
begin
writeln('Please enter the name of the boy :');
readln(nameM[countM]);
countM := countM + 1;
end;
count := count + 1;
end;
if(countM>=0)then
begin
writeln('The boys are:');
for i := 1 to countM do
begin
countM := countM - 1;
writeln(nameM[countM]);
end;
end;
if(countf>=0)then
begin
writeln('The girls are:');
for i := 1 to countF do
begin
countF := countF - 1;
writeln(nameF[countF]);
end;
end;
end.
Before you go
If you have any questions or comments about how to prepare and use the algorithm and trace table for arrays or how to declare and use arrays in the Pascal programming language, be sure to leave them in the comment section below, and we will get back to you in a timely manner.