Last updated on February 14th, 2025 at 04:34 pm

In this article, we explore the for and while loops in Pascal programming. Loops make our lives easier and are great tools in programming when we have many repetitive processes to run.
In this article, we will explore:
- Examples of how to set up and use the for and while loops;
- Writing the for and while loops in both algorithms and Pascal programming; and
- Trace tables of the looping structure.
What are the for and while loops used for?
In Pascal programming, the for and while loops allow us to reduce thousands of lines of code to perform repetitive tasks. A loop repeatedly processes pieces of code until some condition is no longer true.
Example 1 – Loops
Write a program that accepts the names of three students as input, generates an admission number, and prints each child’s name and admission number.
The problem defining diagram
Input | Process | Output |
---|---|---|
childName | 1. Get the childName | admissionNumber |
2. Set the admission number | childName | |
3. Print the admissionNumber and childName. |
Pseudocode of the loop above
Algorithm adNumber;
start
- count = 1
- admissionNumber = 100.
- while(count<=3) start
- a. Display: What is your child’s name
- b. Read: childName
- c. admissionNumber = admissionNumber + 1
- d. Display: admissionNumber
- e. Display: childName
- f. Add count = count + 1
- stop
stop
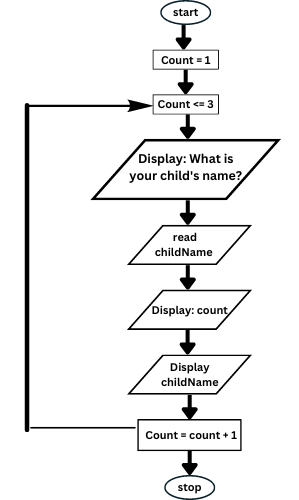
Test data – Names
- Sue Ann
- Misty
- Arlene
Trace table of the loop in example 1
Step | count | admissionNumber | count<=3 | childName | Output |
---|---|---|---|---|---|
1 | 1 | ||||
2 | 100 | ||||
3 | true | ||||
3 a | What is your child’s name? | ||||
3 b | Sue Ann | ||||
3 c | 101 | ||||
3 d | 101 | ||||
3 e | Sue Ann | ||||
3 f | 2 | ||||
3 | true | ||||
3 a | What is your child’s name? | ||||
3 b | Misty | ||||
3 c | 102 | ||||
3 d | 102 | ||||
3 e | Misty | ||||
3f | 3 | ||||
3 | true | ||||
3 a | What is your child’s name? | ||||
3 b | Arlene | ||||
3 c | 103 | ||||
3 d | 103 | ||||
3 e | Arlene | ||||
3 f | 4 | ||||
3 | False |
Example of the Pascal program using the while loop
program adNumber;
var
count : integer;
admissionNumber : integer;
childName : string;
begin
count := 1;
admissionNumber:=100;
while(count<=3)do
begin
writeln('Please enter the name of your child:');
readln(childName);
admissionNumber := admissionNumber + 1;
write(admissionNumber, ' ');
writeln(childName);
count := count + 1;
end;
end.
Loops are often used with control structures and arrays to make our work easier. If you wish to explore control structures, be sure to explore our articles on case statements.
Example 2 – For Loop
Write a program that accepts the names of several employees and their years of service. The program should check their years of service and print the names of all the employees with more than 5 years of service.
The problem defining diagram of example 2
Input | Process | Output |
---|---|---|
names | 1. Read names. | names |
yearsOfService | 2. Read the yearsOfService. | |
3. Find the names of all the persons who have served for more than 5 years. | ||
4. Print names |
The Pseudocode and flowchart of the for loop example 2
Algorithm years
string names
integer yearsOfService
integer count
start
- for(count = 1 to 3) start
- a. Display: Please enter the names of the employees
- b. Read: names
- c. Display: Please enter the years of service
- d. Read yearsOfService
- e. if(yearsOfService>5)
- i Display: names
- stop
stop.
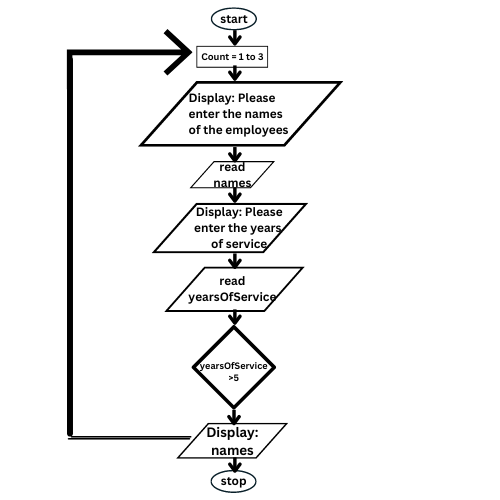
Test data
Names | YearsofService |
---|---|
Aalyah Bowen | 5 |
Louis Smith | 6 |
Jason Lance | 2 |
Trace table of the for loop example 2
Steps | count | Names | YearsOfService | YearsOfService >5 | Output |
---|---|---|---|---|---|
1 | 1 | ||||
1 a | Please enter the names of your employees. | ||||
1 b | Aalyah Bowen | ||||
1 c | Please enter the years of service. | ||||
1 d | 5 | ||||
1 e | false | ||||
1 | 2 | ||||
1 a | Please enter the names of your employees. | ||||
1 b | Louis Smith | ||||
1 c | Please enter the years of service. | ||||
1 d | 6 | ||||
1 e | true | ||||
1 e i | Louis Smith | ||||
1 | 3 | ||||
1 a | Please enter the names of your employees. | ||||
1 b | Jason Lance | ||||
1 c | Please enter the years of service. | ||||
1 d | 2 | ||||
1 e | false |
Pascal program of the for loop – example 2
program yearsServed;
var
names : string;
yearsOfService : integer;
count : integer;
begin
for count := 1 to 3 do
begin
writeln('Please enter the names of the employees:');
readln(names);
writeln('Please enter the years of service of this employee:');
readln(yearsOfService);
if(yearsOfService>3)then
begin
writeln(names);
end;
end;
end.
If you are interested in learning more about the for and while loops, you should explore our article on arrays.
Before you go
We have multiple articles exploring programming in Pascal, which you should explore to become a better Pascal programmer. Be sure to check them out.
We try our best to be as thorough as possible in the information we provide. However, if you have any questions or comments, be sure to leave them in the comment section below, and we will get back to you.