Last updated on August 7th, 2023 at 01:38 am

There are many different computer programming languages. However, if you are new to programming, Pascal is an ideal programming language for a beginner. In this article, we will address:
- What Pascal is;
- Pascal programming software; and
- How to prepare a computer program – from problem-solving to programming.
What is Pascal
Pascal is a high-level procedural programming language. For those of you who do not know what this means, high-level languages (HLL) are machine-independent, meaning they can be compiled and run on different computer systems. They do not require an understanding of the inner workings of the computer. High-level languages are also easy to learn since they closely resemble human language.
Before you begin to code, it is important for you to learn the steps in implementing a computer program and what each entails.
Pascal software
Before you get started programming, you need to download a Pascal compiler and interpreter. There are several available. However, for a beginner programmer, I recommend the following:
- Ezy Pascal;
- Turbo Pascal; or
- This online Pascal compiler.
Some Pascal compilers are available in your Google Play Store for your mobile Android device. The Android version may work a little differently, however.
If you are not planning to use an online compiler, download and install one of the Pascal software above. However, we will use the online software compiler for this series of articles. If you want to learn about Pascal operators, visit our article on Pascal programming operators.
Writing a simple Pascal program
All Pascal programs must have a name (the first line), begin, and end. Here is a simple hello world program as an introduction.
program hello; begin writeln('Hello world!'); end.
The name of your program needs to be descriptive. It should indicate what the program does. If you fail to use a descriptive name, your program will still work. However, there is more to programming than ensuring you have a working program. Using an appropriate name for both your program and variables will make your program easier to code, debug and upgrade.
The program above prints Hello world!. The line program hello indicates that the program is named hello, which is a single descriptive word that indicates what the program does.
Begin and end indicate where the program begins and ends. Writeln, in this instance, indicates that the program will print the information between the brackets to the screen.
Now that we have looked at the most basic Pascal program let’s look at another simple Pascal program with variables. We will look at how to write it using the problem-defining diagram, pseudo code algorithm, trace table, and finally finish with the actual program.
Pascal program example with IPO, algorithm, and trace table
Here is the problem being looked at:
You are asked to write a program that takes in three integer numbers calculate and print the total.
Problem Defining Diagram
Input | Process | Output |
---|---|---|
num1 | 1. Read num1 | total |
num2 | 2. Read num2 | |
num3 | 3. Read num3 | |
4. Calculate the total of the three numbers | ||
5. Print the total |
Algorithm – pseudocode
Algorithm sum
start
- Print: Enter number 1
- Read num1
- Print: Enter number 2
- Read num2
- Print: Enter number 3
- Read num3
- total = num1 + num2 + num3
- Print: total
stop
As you can see in the problem-defining diagram, the items in the process column closely match the algorithm. The main difference is the problem-defining diagram says what is to be done, while the algorithm detail how it is to be done.
For instance, line 4 in the problem-defining diagram says what is to be done (Calculate the total of the three numbers), while the matching line in the algorithm details how it is to be done (total = num1 + num2 + num3).
The trace table
Test Data
- num1 = 34
- num2 = 56
- num3 = 5
Steps | num1 | num2 | num3 | total | Output |
---|---|---|---|---|---|
1. | Enter number 1 | ||||
2. | 34 | ||||
3. | Enter number 2 | ||||
4. | 56 | ||||
5. | Enter number 3 | ||||
6. | 5 | ||||
7. | 95 | ||||
8. | 95 |
Program sum; var num1 : integer; num2 : integer; num3 : integer; total : integer; begin writeln('Please enter the first number:'); readln (num1); writeln('Please enter the second number:'); readln (num2); writeln('Please enter the third number:'); readln (num3); total := num1 + num2 + num3; writeln('The total of the three numbers is:', total); end.
As you can see in this program, there is a section called that begins with var. It contains the list of variables used in the program, and the section after the colon says what type of data the variables will hold. In this instance, it is integer numbers.
If you do not remember what integer numbers are, here are a few examples: -3, -2, -1, 0, 1, 2, 3.
Once the program is written, if you are using the online compiler, simply click execute to run the program. If you are using the Ezy Pascal compiler, you need to compile and run the program. The result is displayed below.
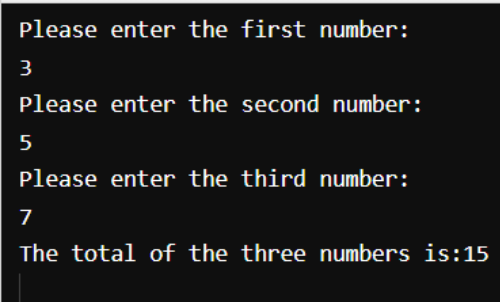
If you wish to learn more about the types of variables used in Pascal and how to declare and initialize them visit our article on Pascal variables.
Before you go
We try our best to be as thorough as possible in the information we provide. However, if you have any questions or comments, please leave them in the section below.