Last updated on October 8th, 2023 at 03:07 pm

The case statement in Pascal is similar to the if-then-else decision-making condition in that it allows you to generate several different answers, which the user’s input will influence. It can be much simpler to implement than the if statement. In this article, we seek to make CSEC students aware of the case decision in Pascal as an alternative to the if statement.
In this article, we will:
- Explain what a case statement is;
- Explore the advantages of using the case statement over the if-then-else statement;
- Explain how the case decision works;
- Algorithms showing the case decision;
- Demonstrate some examples of the case statement in Pascal.
If you are reading this article, there is a good chance that our other lessons may interest you as well. Visit types of errors in programming and Pascal programming operators.
What is the case statement or case decision in programming?
A switch or case statement is a code structure used in programming that allows a program to choose between multiple outcomes based on the user’s input or a flow control statement. It is used as an alternative to the if-then-else or the nested if statement.
Advantages of the case statement in Pascal
Some advantages of using the case decision are:
- The case decision is easier to set up and understand than the if-then-else or nested if statement.
- Because of the simplicity of the syntax used in the case decision, it is also easier to debug.
- Case decisions are much easier to modify than if.
Example of a simple case or switch statement in Pascal programming
program understandCase;
var
month: integer;
message: string;
begin
write('Enter the number of the month in which you were born: ');
readln(month);
case month of
1 : message:='Wow! January girls are awesome. January boys too.';
2 : message:='Wow! You are rather calm and understanding.';
3 : message:='Hmmm! You see right through people and trusts none.';
else message:='Sorry we did not get beyond March. Maybe youd be happy to code the rest.';
end;
writeln(message);
readln;
end.
The result of the above Pascal program
The following is the output of the Pascal program above when 2 is entered as the input.
Enter the number of the month in which you were born: 2
Wow! You are rather calm and understanding.
How does the case decision work?
We will use the above program to explain how the switch statement works.
The value contained in the control statement (month) is evaluated and compared to each value in the case statement (1, 2, 3). If it matches any of the cases, the answer that goes with that match is executed. For instance, if the user entered 2, the message, Wow! You are rather calm and understanding. Arent you?, would be printed to the screen.
Problem example 1 using case
Write a program that takes in a person’s score and prints the corresponding grade.
The problem-defining diagram of the problem above:
Input | Process | Output |
---|---|---|
score | 1. Read the score. | grade |
2. Find the corresponding grade for the score. | ||
3. Print the grade. |
Flow and pseudocode algorithm demonstrating the case decision
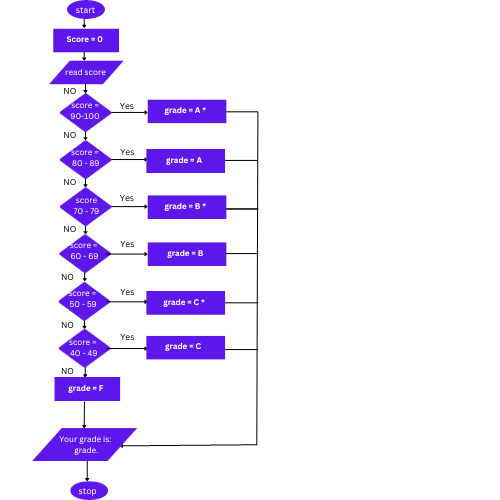
Algorithm studentScore
real score
string grade
start
- score = 0
- Display: Please enter the score.
- Read: score
- Case score
- a. 90 to 100: grade = A+
- b. 80 to 89: grade = A
- c. 70 to 79: grade = B+
- d. 60 to 69: grade = B
- e. 50 to 59: grade = C+
- f. 40 to 49: grade = C
- g. Else grade = F
- stop
- Display: Your grade is: grade;
stop.
Test Data
score = 50;
Trace table of the case decision
In the next section, we will look at the trace table of the case decision for the algorithm above.
Steps | Score | 90 to 100 | 80 to 91 | 70 to 81 | 60 to 71 | 50 to 61 | Output |
---|---|---|---|---|---|---|---|
1 | 0 | ||||||
2 | Please enter the score. | ||||||
3 | 50 | ||||||
4 a. | False | ||||||
4 b. | False | ||||||
4 c. | False | ||||||
4 d. | False | ||||||
4 e. | true | ||||||
5 | Your score is 50. |
Using the case decision in Pascal programming using the algorithm above
program studentScore;
var
score: integer;
grade: string;
begin
score :=0;
writeln('Please enter the score:');
readln(score);
case score of
90..100: grade:= 'A+';
80..89: grade:= 'A';
70..79: grade:= 'B+';
60..69: grade:= 'B';
50..59: grade:= 'C+';
40..49: grade:= 'C';
else grade:= 'F';
end;
writeln('Your grade is ', grade);
readln;
end.
Problem example 2 using a case statement
Write a program that accepts a user’s age as input and finds and prints the school the user is in.
The problem defining diagram
Input | Process | Output |
---|---|---|
age | 1. Read age | school |
2. Find the user’s school. | ||
3. Print the user’s school. |
Flow and pseudocode algorithm of problem 2
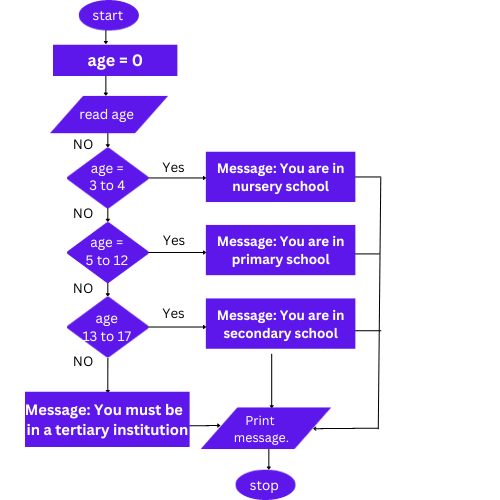
Algorithm usersSchool;
start
- age = 0;
- Display: Please enter your age;
- read: age;
- case age of
- a. 3 to 4 : message = ‘You are in kindergarden.’
- b. 5 to 12 : message = ‘You are in primary school.’
- c. 13 to 17 : message = ‘You are in secondary school.’
- d. Else: message = ‘You must be in at a tertiary institution.’
- stop;
- Display: message;
stop.
Test data
Age = 7
The trace table of example 2 above using the case statement
Steps | age | 3 to 4 | 5 to 12 | Output |
---|---|---|---|---|
1 | 0 | |||
2 | Please enter your age. | |||
3 | 7 | |||
4 a. | False | |||
4 b. | True | |||
6 | You are in primary school. |
Pascal Program showing example 2 using the case statement
program usersSchool;
var
age : integer;
message : string;
begin
age := 0;
writeln('Please enter your age:');
readln(age);
case age of
3..4 : message := 'You are in kindergarden.';
5..12 : message := 'You are in primary school.';
13..17 : message := 'You are in secondary school.';
else message := 'You must be in at a tertiary institution.';
end;
writeln(message);
end.
Before you go
We try to be as thorough as possible in the information we provide. However, if you have any questions or comments, be sure to leave them in the section below, and we will get back to you in a timely manner.