Last updated on September 29th, 2023 at 06:08 pm

Programming operators are symbols that tell a compiler what mathematical or logical manipulation is to be performed on the data entered.
In programming, as in mathematics, there are many operations that may be performed. However, the operators used in mathematics are not all the same as those used in programming. In this article, we will explore:
- The arithmetic operators;
- Boolean operators; and
- Relational operators used in Pascal programming;
- We will also explore what each is used for.
We will also explore what each program operator is used for.
A good programmer does not begin by writing code. Programming is a process that begins with a problem statement followed by the stages in the problem solving process. If you are interested in exploring some tools used in the problem solving process, visit our articles on what is an algorithm and Tracetables.
What are Arithmetic operators in Pascal?
Arithmetic operators are symbols used in the calculation. They tell the computer what process or calculation to perform on the data.
For an example of how these operators work, we will use num1 and num2, where num1 = 9 and num2 = 3.
Programming Operator | Description | Example of use |
---|---|---|
+ | Adds the first and second numbers together. | num1 + num2 = 12 |
– | Subtract the second number from the first. | num1 – num2 = 6 |
* | Multiply the two numbers together. | num1 * num2 = 27 |
/ | Divide the first number by the second. | num1 / num2 = 3 |
Div | Divide the first number by the second. Returns the answer as an integer only. | num1 div num2 = 3 |
Mod | Mod returns the remainder in a division. | num1 mod num2 = 0 |
More on mod and div programming operators
When mod is used, the program ignores the integer in the answer and prints the remainder only. For instance, if we were to use num1 mod num2 where num1 holds 7 and num2 holds 2, the answer would be 3 and the remainder 1. So num1 mod num2 would return 1 as the answer.
On the other hand, div is used to divide one number by another when the answer is to be given as an integer number only. So using the values stored in num1 and num2 above, the expression would look like this num1 div num2, and the answer would be returned as 3 with the remainder ignored.
Example of these arithmetic operators in use in a Pascal program
In the following program, we demonstrate the use of these arithmetic operators:
Program Operators; var num1 : integer; num2 : integer; sum : integer; mul : integer; sub : integer; quotient : real; quotient2 : integer; remainder : integer; begin num1 := 5; num2 := 3; sum := num1 + num2; writeln('The total of number 1 and number 2 is: ', sum, '.'); sub := num1 - num2; writeln('The difference of number 1 - number 2 is: ', sub, '.'); mul := num1 * num2; writeln('The product of number 1 and number 2 is: ', mul, '.'); quotient := num1/num2; writeln('The quotient of number 1 divided by number 2 is: ', quotient:2:2, '.'); remainder := num1 mod num2; writeln('The remainder of number 1 divided by number 2 is: ', remainder, '.'); quotient2 := num1 div num2; writeln('The remainder of number 1 divided by number 2 is: ', quotient2, '.'); end.
All novice programmers need to debug their programs from time to time. However, identifying and correcting errors calls for good knowledge of error checking. For this, you should visit our article on the types of errors in programming.
The result of the program named operators above is
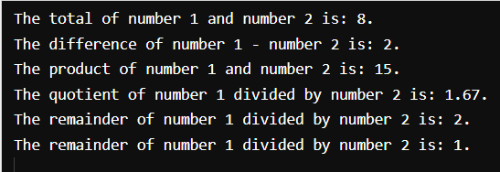
If you are new to programming, you should also explore our article on programming fundamentals – variables vs. consonants to learn about what are variables and consonants, their types, and their uses.
What are the boolean operators (program operators) used in Pascal programming
Boolean operators are expressions that, when used in programming, return the results as true or false only. In the table below, we list these operators, their descriptions, and examples of their use. For this demonstration, I will use the value1, value2, value3, and value4 where
- value1 holds true;
- value2 holds true;
- value3 holds false; and
- value4 holds false.
Boolean operator | Description | Example of use |
---|---|---|
AND | This operation reads true when both of the variables hold the value true. | value1 AND value2 = true |
AND | This operation reads false when one variable holds the value true, and the other holds the value false. | value1 AND value3 = false |
AND | This operation reads false when both variables hold the value false. | (value3 AND value4) |
NOT | This operation reads false where both variables hold the value true. | NOT (value1 AND value2) = false |
NOT | This operation reads true when one variable holds the value true, and the other holds false. | NOT (value1 AND value3) = true |
NOT | This operation reads true when both variables hold the value false. | NOT (value3 AND value4) = true |
OR | This operation reads true when both variables hold the value true. | value1 OR value2 = true |
OR | The operation reads true when only one variable holds the value true. | value1 OR value3 = true |
OR | The operation reads false when only both variables hold the value false. | value3 OR value4 = false |
Example of these Boolean operators in use in a program
Program booleanOperators; var first : boolean; second : boolean; third : boolean; fourth : boolean; begin first := true; second := true; third := false; fourth := false; if(first AND second)then writeln('1. For the boolean operator AND, first and second reads reads true.'); if(second AND third)then writeln('2. For the boolean operator AND, second and third reads true.'); if(third AND fourth)then writeln('3. For the boolean operator AND, third and fourth reads true.'); if(first OR second)then writeln('4. For the boolean operator OR first and second reads true.'); if(first OR third)then writeln('5. For the boolean operator OR first and third reads true.'); if(third OR fourth)then writeln('6. For the boolean operator OR third and fourth reads true.'); if NOT(first AND second)then writeln('7. For the boolean operator NOT first and second reads true.'); if NOT(first AND third)then writeln('8. For the boolean operator NOT first and third reads true.'); if NOT(third AND fourth)then writeln('9. For the boolean operator NOT third and fourth reads true.'); end.
Result of the program using Boolean operators above
The image below is the result of the above program
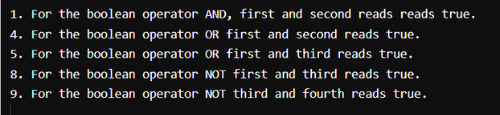
What are relational operators
Relational operators are one of the most useful programming operators. They are used when we compare values. In the table below, we look at the relational operators used in Pascal programming, examples of their use, and a description of what happens when we use them.
For this demonstration, we will use value5 and value6, where
- value5 holds 8; and
- value6 holds 3.
Relational Operator | Description | Example of use |
---|---|---|
> | This operator compares the value held by two variables to see if the first is bigger than the second. | if(value5 > value6) evaluates to true |
< | This operator compares the value held by two variables to see if the first is smaller than the second. | if(value5 < value6) evaluates to false |
= | This operator indicates that a check should be made to see if the first and second values are equal. | if(value5 = value6) evaluates to false |
<> | This operator checks to see if the first and second variables are unequal. | if(value5 <> value6) evaluates to true |
>= | This operator checks to see if the first value is greater than or equal to the second. | if(value5 >= value6) evaluates to true |
<= | This operator checks to see if the first value is smaller than or equal to the second. | if(value5 <= value6) evaluates to false |
Would you like to learn how to deal with decision-making in Pascal programming? Visit our article on the if statement and the case structure to learn more.
Example of relational operators in use in Pascal
program relationalOperators; var value1 : integer; value2 : integer; value3 : integer; value4 : integer; begin value1 := 8; value2 := 7; value3 := 8; if(value1>value2)then writeln('1. Value one is greater.') else writeln('2. Value two is greater.'); if(value1<value2)then writeln('3. Value 1 is amaller than value two.') else writeln('4. Value two is smaller than value1.'); if(value1=value3)then writeln('5. Value one and three are equal.') else if(value1=value2)then writeln('6. Value one and two are equal.'); if(value1>=value2)then writeln('7. Value 1 is greater than or equal to value 2.') else if(value1<=value2)then writeln('8. Value1 is less than or equal to value 2.'); if(value1<>value2)then writeln('9. Value1 and 2 are unequal.'); end.
Result of the program above using relational operators (program operators)
This is an example of the result of the program using the relational operators above.
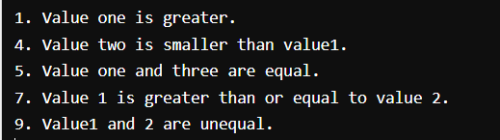
Exercise
The table below is based on the information on operators above. 1 represents true, while 0 represents false. Fill in the rightmost column with T for true or F for false where applicable.
Operator | A | B | True/False |
---|---|---|---|
AND | 1 | 1 | |
AND | 1 | 0 | |
AND | 0 | 1 | |
AND | 0 | 0 | |
OR | 1 | 1 | |
OR | 1 | 0 | |
OR | 0 | 1 | |
OR | 0 | 0 | |
NOT(A AND B) | 1 | 1 | |
NOT(A AND B) | 1 | 0 | |
NOT(A AND B) | 0 | 1 | |
NOT(A AND B) | 0 | 0 |
Before you go
We do our best to be as thorough as possible in the information we provide. However, if you have any questions or comments, be sure to leave them in the section below, and we will get back to you in a timely manner.